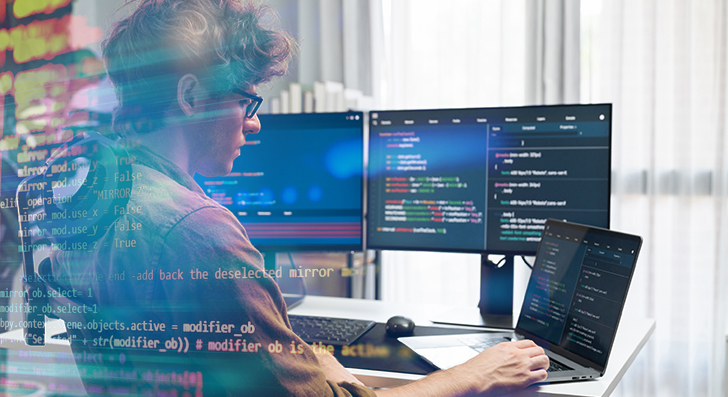
Scalability implies your software can cope with progress—a lot more users, far more info, and much more traffic—without the need of breaking. Being a developer, developing with scalability in your mind saves time and worry afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on later—it ought to be component of your respective strategy from the start. Numerous apps fail if they increase quick mainly because the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your procedure will behave under pressure.
Start out by creating your architecture to get adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. Rather, use modular layout or microservices. These styles break your app into scaled-down, unbiased parts. Every single module or assistance can scale By itself with no influencing the whole method.
Also, think of your databases from working day one. Will it want to manage 1,000,000 customers or perhaps 100? Select the ideal type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t require them but.
One more significant place is to stay away from hardcoding assumptions. Don’t write code that only works under present situations. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that assistance scaling, like concept queues or function-driven methods. These assist your app handle extra requests without the need of having overloaded.
After you Make with scalability in your mind, you are not just planning for achievement—you're reducing future problems. A properly-planned method is simpler to maintain, adapt, and mature. It’s superior to organize early than to rebuild later.
Use the Right Databases
Selecting the correct databases can be a critical Portion of developing scalable apps. Not all databases are crafted the exact same, and using the Mistaken one can gradual you down or simply lead to failures as your application grows.
Get started by knowledge your info. Is it really structured, like rows in the table? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. They are potent with relationships, transactions, and regularity. Additionally they support scaling approaches like study replicas, indexing, and partitioning to manage much more website traffic and info.
In the event your info is a lot more flexible—like person activity logs, merchandise catalogs, or files—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and produce designs. Are you undertaking many reads with fewer writes? Use caching and browse replicas. Will you be handling a large produce load? Look into databases that could deal with substantial produce throughput, or even function-dependent details storage methods like Apache Kafka (for short term data streams).
It’s also wise to Consider ahead. You may not need to have Highly developed scaling attributes now, but selecting a database that supports them suggests you received’t need to switch later.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your data depending on your access patterns. And usually check database efficiency while you expand.
In a nutshell, the ideal databases will depend on your application’s framework, pace wants, And the way you count on it to expand. Get time to pick wisely—it’ll conserve plenty of difficulty later.
Improve Code and Queries
Speedy code is vital to scalability. As your app grows, each little hold off provides up. Inadequately published code or unoptimized queries can decelerate efficiency and overload your program. That’s why it’s crucial to build economical logic from the beginning.
Commence by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything at all unnecessary. Don’t pick the most intricate Answer if a straightforward one particular operates. Keep the features brief, concentrated, and simple to check. Use profiling applications to search out bottlenecks—areas where by your code can take as well extensive to run or uses an excessive amount memory.
Subsequent, evaluate your database queries. These normally gradual issues down much more than the code itself. Be certain Each and every question only asks for the data you really need. Prevent SELECT *, which fetches anything, and rather select certain fields. Use indexes to hurry up lookups. And steer clear of executing a lot of joins, Specifically throughout large tables.
In case you see the identical facts being asked for many times, use caching. Shop the final results quickly utilizing equipment like Redis or Memcached therefore you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. As opposed to updating a row one after the other, update them in groups. This cuts down on overhead and tends to make your app far more economical.
Make sure to test with huge datasets. Code and queries that operate high-quality with a hundred documents might crash once they have to deal with one million.
To put it briefly, scalable applications are speedy applications. Keep your code limited, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of extra buyers and more traffic. If everything goes through 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these tools help keep the application rapidly, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the perform, the load balancer routes customers to various servers depending on availability. This means no one server will get overloaded. If 1 server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data quickly so it may be reused swiftly. When users ask for the identical info all over again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You could serve it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) merchants data in memory for rapid access.
two. Client-aspect caching (like browser caching or CDN caching) stores static information near the consumer.
Caching cuts down database load, enhances speed, and will make your app additional efficient.
Use caching for things which don’t improve usually. And normally make certain your cache is up-to-date when data does click here adjust.
To put it briefly, load balancing and caching are straightforward but impressive resources. Alongside one another, they help your app cope with more end users, continue to be fast, and Recuperate from challenges. If you propose to develop, you require both of those.
Use Cloud and Container Resources
To create scalable apps, you need resources that permit your application grow effortlessly. That’s the place cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and solutions as you will need them. You don’t really need to buy hardware or guess future capacity. When visitors raises, you'll be able to incorporate far more methods with just a couple clicks or routinely working with car-scaling. When website traffic drops, you may scale down to save cash.
These platforms also present companies like managed databases, storage, load balancing, and stability applications. You could center on making your application as an alternative to handling infrastructure.
Containers are An additional important tool. A container offers your application and every little thing it has to run—code, libraries, configurations—into one particular unit. This makes it simple to maneuver your application among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes assist you to regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your respective application crashes, it restarts it immediately.
Containers also enable it to be very easy to individual areas of your app into expert services. It is possible to update or scale parts independently, which is perfect for overall performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quickly, deploy very easily, and Recuperate promptly when issues transpire. If you'd like your application to develop devoid of limits, commence applying these resources early. They help save time, decrease chance, and help you remain centered on setting up, not fixing.
Keep an eye on Everything
For those who don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your application is performing, spot troubles early, and make superior decisions as your app grows. It’s a crucial Section of setting up scalable systems.
Commence by tracking primary metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—observe your application much too. Keep an eye on how long it takes for customers to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a services goes down, you should get notified immediately. This helps you take care of challenges rapid, typically ahead of consumers even discover.
Checking is likewise handy if you make adjustments. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it will cause actual damage.
As your application grows, targeted traffic and information maximize. Devoid of monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring aids you keep the app responsible and scalable. It’s not almost recognizing failures—it’s about comprehension your program and making sure it really works effectively, even stressed.
Last Feelings
Scalability isn’t just for massive businesses. Even smaller apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish applications that grow easily without the need of breaking under pressure. Start off compact, Feel major, and build wise.